Sui TypeScript SDK 简明教程
在本教程中,我将介绍如何通过 TypeScript 与 Sui 网络进行交互。我们将学习如何从 TypeScript 程序读取区块链的状态、将交易写入链中。
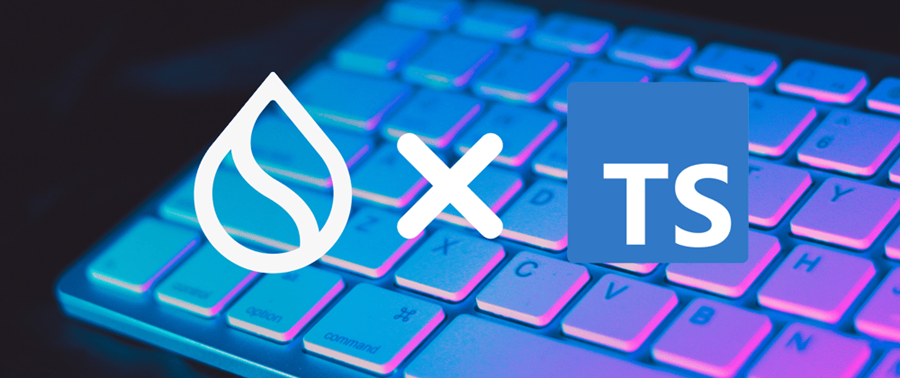
一键发币: SUI | SOL | BNB | ETH | BASE | ARB | OP | POLYGON | 跨链桥/跨链兑换
Sui 是当下最流行的区块链,尽管 Move 是编写 Sui 智能合约的圣杯,但对 TypeScript 的支持也很重要。你可以使用 TypeScript 与 Sui 以及生态系统中的大多数 DeFi 应用进行交互和使用。
在本教程中,我将介绍如何通过 TypeScript 与 Sui 网络进行交互。我们将学习如何从 TypeScript 程序读取区块链的状态、将交易写入链中。
1、Sui 和 TypeScript 入门
唯一的先决条件是你需要基本的 JS/TS 知识才能顺利运行本教程。我会带你完成其他所有事情。
首先,在你的终端中创建一个新的 TypeScript 项目并初始化一个新的 Node.js 项目:
mkdir SuiTS
cd SuiTS
npm init -y
如果你还没有安装 TypeScript,请将其安装为开发依赖项:
npm install typescript --save-dev
npm install ts-node //runs TS without the need for transpiling
现在,你可以初始化一个新的 TypeScript 项目。此命令将创建一个 tsconfig.json
文件,其中包含你可以为项目自定义的默认选项:
npx tsc --init
打开 tsconfig.json
并粘贴这些配置:
{
"compilerOptions": {
"target": "ES2020",
"module": "CommonJS",
"outDir": "./dist",
"rootDir": "./src",
"strict": true,
"esModuleInterop": true,
"types": ["node"],
"resolveJsonModule": true
},
"exclude": ["node_modules"],
"scripts": {
"build": "tsc",
"start": "node dist/index.js"
}
}
创建一个 src
目录,你将在其中添加 TypeScript 文件:
mkdir src
touch src/index.ts
最后,使用此命令安装 Sui TypeScript SDK:
npm i @mysten/sui.js
现在已全部设置完毕。我们可以开始编写与 Sui 区块链交互的 TypeScript 程序。
2、连接到 Sui 区块链
我们必须连接到 Sui 区块链才能与链交互。
首先,从 SDK 客户端模块导入 getFullnodeUrl
和 SuiClient
:
import { getFullnodeUrl, SuiClient } from '@mysten/sui/client';
现在,根据所需的连接,你可以使用 getFullnodeUrl
检索 Sui 测试网、主网、本地网或开发网的完整节点 URL;然后,使用 SuiClient
连接到客户端实例:
import { getFullnodeUrl, SuiClient } from '@mysten/sui/client';
const rpcUrl = getFullnodeUrl('mainnet');
const client = new SuiClient({ url: rpcUrl });
要测试你的连接,可以使用 getLatestSuiSystemState
检索网络的最新状态:
// index.ts
import { getFullnodeUrl, SuiClient } from '@mysten/sui/client';
const rpcUrl = getFullnodeUrl("mainnet");
const client = new SuiClient({ url: rpcUrl });
async function getNetworkStatus() {
const currentEpoch = await client.getLatestSuiSystemState();
console.log(currentEpoch)
}
getNetworkStatus();
现在,将 TypeScript 代码转换为 JavaScript 并使用以下命令运行它:
ts-node index.ts
执行命令时,你应该会看到类似这样的输出。
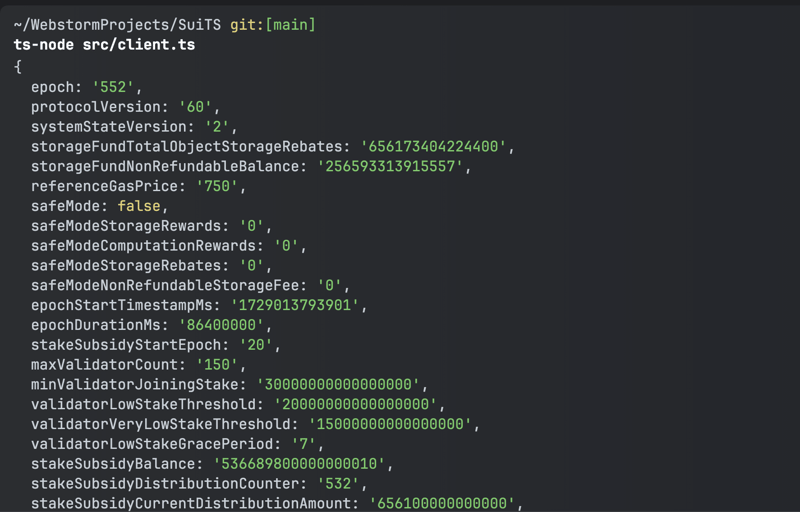
3、创建 Sui 钱包
创建钱包是另一种流行的操作,如果你在 Sui 网络上构建,这可能会很方便。
以下是如何生成 Sui 钱包密钥对并从密钥对中检索私钥和公钥:
import { Ed25519Keypair } from '@mysten/sui/keypairs/ed25519';
import { getFullnodeUrl, SuiClient } from '@mysten/sui/client';
const rpcUrl = getFullnodeUrl("mainnet");
const client = new SuiClient({ url: rpcUrl });
// random Keypair
const keypair = new Ed25519Keypair();
const publicKey = keypair.getPublicKey();
const privatekey = keypair.getSecretKey();
console.log(privatekey.toString());
console.log(publicKey.toSuiAddress());
Ed25519Keypair
函数创建一个新的密钥对。 getPublicKey
和 getPrivateKey
方法分别允许你访问公钥和私钥。
以下是我使用该程序生成的私钥和公钥的字符串输出:
suiprivkey1qq9r6rkysny207t5vr7m5025swh7w0wzra9p0553paprhn8zshqsx2rz64r
New Sui Address: 0xbd46d7582ced464ef369114252704b10317436ef70f196a33fcf2c724991fcba
我将为该钱包注入 0.25 Sui,以进行下一组操作。请随意验证和扫描钱包。不要发送任何资金;这只是一个虚拟钱包。
4、读取 Sui 钱包余额
可以使用客户端实例的 getCoins
方法来检索钱包地址中Sui币的详细信息:
import { getFullnodeUrl, SuiClient } from '@mysten/sui/client';
// use getFullnodeUrl to define the Devnet RPC location
const rpcUrl = getFullnodeUrl('mainnet');
// create a client connected to devnet
const client = new SuiClient({ url: rpcUrl });
async function getOwnedCoins() {
const coins = await client.getCoins({
owner: '0xbd46d7582ced464ef369114252704b10317436ef70f196a33fcf2c724991fcba',
});
console.log(coins);
}
getOwnedCoins();
该函数仅返回有关 Sui 币的详细信息和详细信息。输出单位是 MIST,即 Sui gas 代币。 1 SUI 等于 10 亿 MIST:
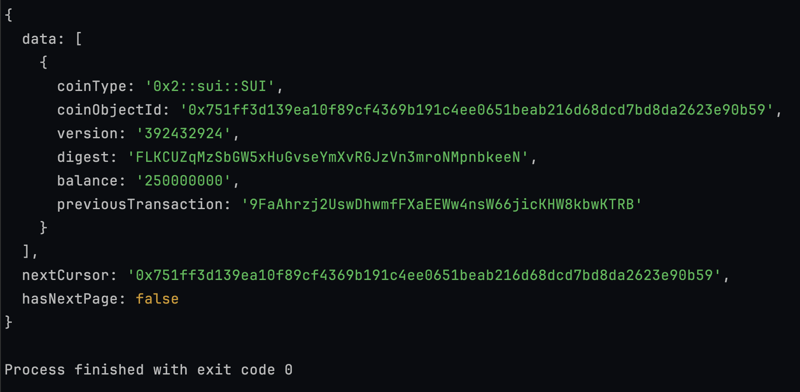
getAllCoins
方法 可以以相同的方式用于获取钱包中所有加密币的列表:
async function getAllCoins() {
// Get the list of owned coins (tokens) for the given owner address
const ownedCoins = await client.getAllCoins({ owner: "0xbd46d7582ced464ef369114252704b10317436ef70f196a33fcf2c724991fcba" });
// Access the coin data
const coins = ownedCoins.data;
// Iterate through the coins and print their details
for (const coin of coins) {
console.log(`Coin Type: ${coin.coinType}`);
console.log(`Coin Object ID: ${coin.coinObjectId}`);
console.log(`Balance: ${coin.balance}`);
console.log('--------------------');
}
// If there is more data, handle pagination
if (ownedCoins.hasNextPage) {
console.log('More data available. Fetching next page...');
// You can handle the next page using ownedCoins.nextCursor if needed
}
}
getAllCoins();
对于此示例,我在 Hop Aggregator 上用一些 Sui 换取了 $FUD,这是运行程序后的输出:
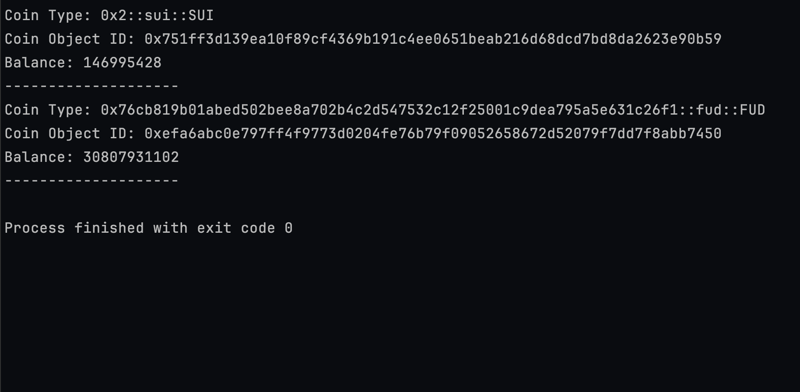
5、发送加密币或对象
最后,有趣的部分是我们将学习如何在区块链上发送交易。
让我们将一些 $FUD 代币发送到另一个钱包。这适用于 Sui 网络上的任何加密币:
import {getFullnodeUrl, SuiClient} from '@mysten/sui/client';
import {Ed25519Keypair} from '@mysten/sui/keypairs/ed25519';
import {Transaction} from '@mysten/sui/transactions';
// Set the RPC URL to connect to the Sui mainnet
const rpcUrl = getFullnodeUrl("mainnet");
const client = new SuiClient({url: rpcUrl});
// Create the keypair using the private key
const keypair = Ed25519Keypair.fromSecretKey("suiprivkey1qq9r6rkysny207t5vr7m5025swh7w0wzra9p0553paprhn8zshqsx2rz64r");
// FUD coin type
const FUD_TYPE = '0x76cb819b01abed502bee8a702b4c2d547532c12f25001c9dea795a5e631c26f1::fud::FUD';
async function sendFUD() {
const tx = new Transaction();
// Fetch FUD coins owned by the sender
const coins = await client.getCoins({owner: keypair.getPublicKey().toSuiAddress(), coinType: FUD_TYPE});
if (coins.data.length === 0) {
console.log("No FUD coins found in the wallet.");
return;
}
// Choose the first available FUD coin and split it for the transfer (adjust amount if needed)
const [coin] = tx.splitCoins(coins.data[0].coinObjectId, [100000000]);
tx.transferObjects([coin], '0xb0042cf2c5a16d0a240fc1391d570cd5fe06548f860583f1878c327db70f2a22');
const result = await client.signAndExecuteTransaction({signer: keypair, transaction: tx});
await client.waitForTransaction({digest: result.digest});
console.log("Transaction successful. Digest:", result.digest);
}
sendFUD().then(console.log).catch(console.error);
首先,我检查钱包中是否有一些 $FUD,并将其拆分以进行转账。 tx.transferObjects
将拆分的硬币转移到指定的地址。
最后,我们需要使用 client.signAndExecuteTransaction
签署交易,然后可以使用 waitForTransaction
等待交易以确认交易已完成
6、结束语
你已经学会了使用官方 TypeScript SDK 与 Sui 区块链进行交互,可以使用新获得的知识在 Sui 上构建很多东西,例如构建钱包和机器人。
接下来,可以通过学习如何与 Sui 上的 Move 合约交互来构建更复杂的 dApp,从而进一步了解这一点
原文链接:How to Use the Sui TypeScript SDK
DefiPlot翻译整理,转载请标明出处
免责声明:本站资源仅用于学习目的,也不应被视为投资建议,读者在采取任何行动之前应自行研究并对自己的决定承担全部责任。