Python发送Solana交易
在本教程中,我们将了解如何使用 Python 在 Solana 区块链中发送交易! 我们将使用 Solathon,一个易于使用的Solana Python SDK。
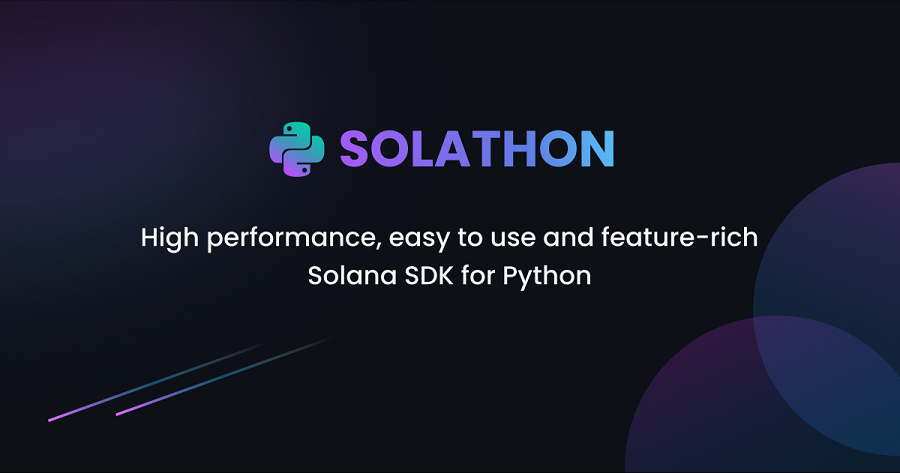
一键发币: SUI | SOL | BNB | ETH | BASE | ARB | OP | POLYGON | 跨链桥/跨链兑换
在本教程中,我们将了解如何使用 Python 在 Solana 区块链中发送交易!
为此,你不需要了解任何复杂性或幕后发生的事情。 我们将使用 Solathon,这是一个易于使用、功能丰富的 Python SDK,适用于 Solana。
确保已安装 Python 3.7 或更高版本,现在使用以下命令安装包:
pip install solathon
首先,我们需要创建一个客户端,用于与 Solana 的 JSON RPC 交互。 出于测试目的,我们将使用 devnet,因为它不需要你拥有真正的 SOL 平衡。 以下代码在 devnet 上创建一个客户端以及我们稍后需要的所有导入:
from solathon.core.instructions import transfer
from solathon import Client, Transaction, PublicKey, Keypair
client = Client("https://api.devnet.solana.com")
现在,我们需要定义三件事; 首先是发送交易的账户,第二是接收交易的账户,第三是我们想要在 lamports 中发送的金额(这是 Solana 区块链上交易金额的标准单位)。 看看下面的代码:
sender = Keypair.from_private_key("you_private_key_here")
receiver = PublicKey("receiver_public_key")
amount = 100
在这里,发送者需要是一个密钥对,它是公钥和私钥的组合,你需要从钱包中获取私钥才能初始化 Keypair 对象。 然后我们需要我们希望将交易发送到的帐户的公钥,最后我们有了要在lamports中转移的金额。 你可以使用Solaton提供的 sol_to_lamport
函数来轻松转换。
现在,每笔交易都需要定义一些需要执行的指令,这里我们只需要一条指令,那就是转账:
instruction = transfer(
from_public_key=sender.public_key,
to_public_key=receiver,
lamports=100
现在,我们需要使用指令和签名者列表(在本例中只是发送者)来初始化交易对象:
transaction = Transaction(instructions=[instruction], signers=[sender])
最后我们可以发送此交易并打印结果:
result = client.send_transaction(transaction)
print("Transaction result: ", result)
这是完整的代码:
from solathon.core.instructions import transfer
from solathon import Client, Transaction, PublicKey, Keypair
client = Client("https://api.devnet.solana.com")
sender = Keypair.from_private_key("your_private_key")
receiver = PublicKey("receiver_public_key")
amount = 100
instruction = transfer(
from_public_key=sender.public_key,
to_public_key=receiver,
lamports=100
)
transaction = Transaction(instructions=[instruction], signers=[sender])
result = client.send_transaction(transaction)
print("Transaction response: ", result)
你可以查看官方文档以了解交易发送的更多详细信息。
原文链接:How to send Solana transactions using Python
DefiPlot翻译整理,转载请标明出处
免责声明:本站资源仅用于学习目的,也不应被视为投资建议,读者在采取任何行动之前应自行研究并对自己的决定承担全部责任。