用LLM预测Crypto市场情绪
本文介绍如何使用 LLM 和一些提示工程来从财经新闻中提取加密市场信号。
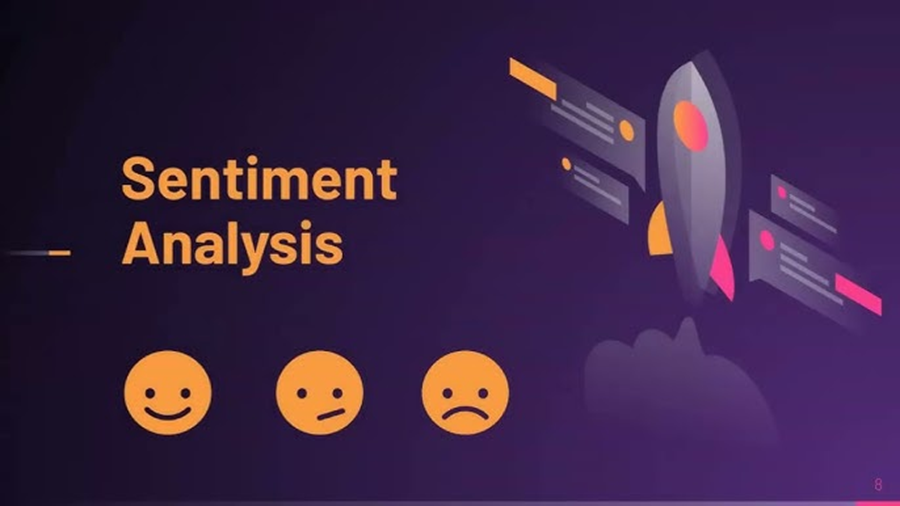
一键发币: SUI | SOL | BNB | ETH | BASE | ARB | OP | POLYGON | AVAX | FTM | OK
假设你想构建一个 ML 服务,从财经新闻中提取加密市场信号。此类服务的输出可以与其他预测功能(如所有货币的实时市场价格)一起输入到预测 ML 模型中,以得出最佳的价格预测。
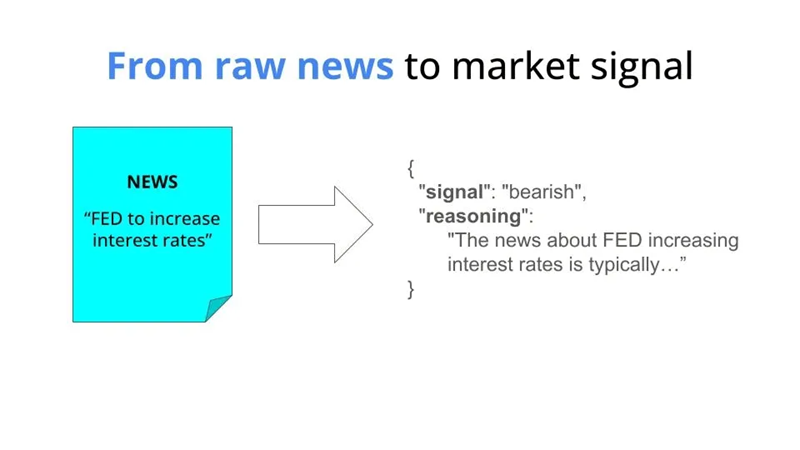
让我向你展示如何使用 LLM 和一些提示工程来解决这个现实世界的问题。
1、问题
我们想要构建一个 Python 微服务,
给定一个新闻标题:
news = "FED to increase interest rates"
输出市场信号和这个分数背后的原因:
{
"signal": "bearish",
"reasoning": "The news about FED increasing interest rates is typically bearish for crypto markets for several reasons:\n1. Higher interest rates make borrowing more expensive, reducing liquidity in the market\n2. Higher rates make traditional yield-bearing investments more attractive compared to crypto\n3. Risk assets like cryptocurrencies tend to perform poorly in high interest rate environments\n4. Historically, crypto prices have shown negative correlation with interest rate hikes"
}
信号是一个分类信号,有 3 个可能值:
- bullish:看涨(对市场产生积极影响)
- neutral:中性(中性/不明确的影响)或
- bearish:看跌(对市场产生负面影响)
将原始输入转换为结构化输出是 LLM 擅长的事情,因此我们在这里尝试一下是有意义的。
让我向您展示如何使用强大的 LLM 和一些提示工程在 Python 中实现这一点。
你可以在此存储库中找到所有源代码。
2、解决方案
我们希望我们的模型能够提取原始文本数据并输出结构化响应。
我知道解决这个问题的最快方法是:
- 使用具有函数调用功能的强大 LLM(例如 Claude)和
- 像 llama index 这样的库,以确保输出具有所需的格式。
在这个例子中,我将使用 Antrhopic 的 Claude,但你可以随意将其替换为其他 LLM,例如OpenAI,或使用 Ollama 本地运行的 Llama 3.2。
如果你想跟着我一起做,需要在这里获取你的 API 密钥并花费更少的 0.01 美元。
这些是构建它的步骤。
3、引导项目
我开始使用 uv 作为我的 Python 构建工具。如果你还没有,我建议你试一试。
在 Linux/Mac 上安装 uv:
curl -LsSf https://astral.sh/uv/install.sh | sh
或在 Windows 上:
powershell -ExecutionPolicy ByPass -c "irm https://astral.sh/uv/install.ps1 | iex"
然后使用此命令从命令行引导你的项目:
uv init crypto-sentiment-extractor --lib
cd crypto-sentiment-extractor
4、安装 Python 依赖项
即llama-index 和 llama-index-llms-anthropic 以便轻松与 Claude 交互,并控制输出格式:
uv add llama-index-llms-anthropic
uv add llama-index
使用pydantic 以类型验证增强我们的 Python 代码:
uv add pydantic
使用pydantic-settings 以轻松加载和验证配置参数:
uv add "pydantic-settings>=2.6.1"
5、设置配置
使用你的 API 密钥和其他配置创建一个 anthropic.env
文件参数:
API_KEY="YOUR_API_GOES_HERE"
MAX_TOKENS=300
MODEL_NAME=claude-3-5-sonnet-20241022
并使用此 pydantic-settings 类轻松将它们加载到你的 Python 运行时中:
from pydantic_settings import BaseSettings, SettingsConfigDict
class AnthropicConfig(BaseSettings):
model_config = SettingsConfigDict(env_file='anthropic.env', env_file_encoding='utf-8')
api_key: str
model_name: str = "claude-3-opus-20240229"
max_tokens: int = 300
anthropic_config = AnthropicConfig()
6、使用 Pydantic 定义 LLM 输出
我们创建一个 pydantic 类,其中包含我们希望 LLM 输出的字段名称和描述:
from typing import Literal
from pydantic import BaseModel, Field
class NewsMarketSignal(BaseModel):
"""
Market signal of a news article about the crypto market
"""
signal: Literal["bullish", "bearish", "neutral"] = Field(description="""
The market signal of the news article about the crypto market.
Set it as neutral if the text is not related to crypto market, or not enough
information to determine the signal.
""")
reasoning: str = Field(description="""
The reasoning for the market signal.
Set it as non-relevant if the text is not related to crypto market
Set it as non-enough-info if there is not enough information to determine the signal
""")
添加字段描述非常重要。这些提供了您的 LLM 有效解决任务所需的上下文。
7、定义提示模板
此系统提示指示语言模型的预期行为和功能。例如:
self.prompt = PromptTemplate(
"""
You are an expert at analyzing news articles related to cryptocurrencies and extracting market signal from the text in a structured format.
Here is the news article:
{text}
""")
8、创建与LLM交互的客户端对象
from .config import AnthropicConfig
config = AnthropicConfig()
from llama_index.llms.anthropic import Anthropic
self.llm = Anthropic(
model=config.model_name,
max_tokens=config.max_tokens,
api_key=config.api_key,
)
9、向LLM 发送请求
我们想要:
- 生成 NewsMarketSignal 类中指定的结构化输出,
- 使用系统提示 self.prompt,
- 对于给定的新闻文本
response = self.llm.structured_predict(
NewsMarketSignal, self.prompt, text=text
)
你可以使用自定义 Python 对象封装和组织此逻辑:
# claude.py
class ClaudeMarketSignalExtractor:
"""
A class to extract market signal from text using Claude's API
"""
def __init__(self):
"""
Initialize the ClaudeMarketSignalExtractor.
"""
config = AnthropicConfig()
self.llm = Anthropic(
model=config.model_name,
max_tokens=config.max_tokens,
api_key=config.api_key,
)
self.prompt = PromptTemplate(
"""
You are an expert at analyzing news articles related to cryptocurrencies and
extracting market signal from the text in a structured format.
Here is the news article:
{text}
"""
)
def get_signal(self, text: str) -> NewsMarketSignal:
"""
Extract the market signal from the text.
Args:
text (str): The text to extract the sentiment from.
Returns:
SentimentScore: The sentiment score.
"""
response = self.llm.structured_predict(
NewsMarketSignal, self.prompt, text=text
)
return response
最后,要查看实际效果,请运行:
uv run python -m crypto_sentiment_extractor.claude
例如:
Trump Said appoints Crypto Lawyer Teresa Goody Guillén to Lead SEC
{
"signal": "bullish",
"reasoning": "The appointment of Teresa Goody Guill\u00e9n, a crypto lawyer, to lead the SEC under Trump's potential administration is likely bullish for the crypto market. This is significant because:\n1. Having a crypto lawyer in charge of the SEC suggests a more crypto-friendly regulatory approach\n2. This could potentially lead to more favorable policies and regulations for the cryptocurrency industry\n3. The appointment of someone with crypto expertise indicates a shift from the current SEC's relatively strict stance on crypto\n4. This could potentially lead to clearer regulatory frameworks and better institutional adoption"
}
FED to increase interest rates
{
"signal": "bearish",
"reasoning": "The news about FED increasing interest rates is typically bearish for crypto markets because:\n1. Higher interest rates make risk assets like cryptocurrencies less attractive to investors\n2. It reduces market liquidity as borrowing becomes more expensive\n3. Historically, crypto prices have shown negative correlation with interest rate hikes\n4. Investors tend to move capital from speculative assets to safer yield-bearing instruments during rate hike cycles"
}
The grass is green
{
"signal": "neutral",
"reasoning": "The text \"The grass is green\" is completely unrelated to the cryptocurrency market or any financial markets. It's a simple statement about nature/landscaping. Therefore, there is no relevant market signal to extract."
}
10、下一步计划
我们构建的是一个概念证明。这意味着它“似乎有效”,但这还不够。
为了使其以稳健的方式工作,你需要评估这些输出的质量。但这是我们将留到另一天的事情。
原文链接:Crypto sentiment with LLMs
DefiPlot翻译整理,转载请标明出处
免责声明:本站资源仅用于学习目的,也不应被视为投资建议,读者在采取任何行动之前应自行研究并对自己的决定承担全部责任。